[DSA] Introduction about Data Structure and Algorithm
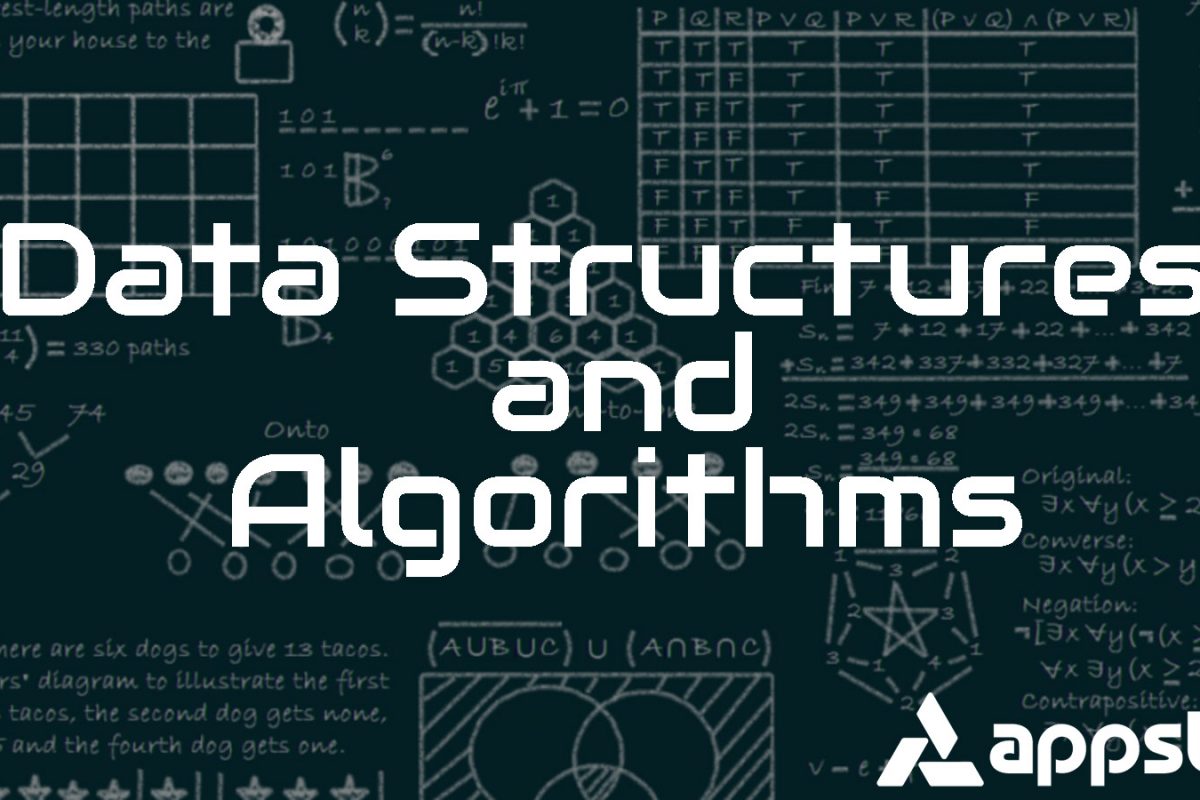
1. Algorithms:
An algorithm is a step-by-step procedure that is designed to solve a specific problem. Algorithms can be expressed in many forms, including flowcharts, programming code, and natural language. Algorithms can be used to perform various tasks, such as sorting data, searching for information, and solving mathematical problems. The quality of an algorithm is judged by its correctness, efficiency, clarity, and robustness.
Example:
- a. Bubble Sort Algorithm: This algorithm is used for sorting an array of elements. It repeatedly compares adjacent elements and swaps them if they are in the wrong order until the array is sorted.
- b. Binary Search Algorithm: This algorithm is used for searching a sorted array. It repeatedly divides the array in half until the target element is found.
- c. Dijkstra's Algorithm: This algorithm is used for finding the shortest path between two nodes in a graph. It uses a priority queue to keep track of the next node to visit.
2. Pseudo code:
Pseudo code is a high-level description of an algorithm that is written in plain English or any other natural language. It is used to describe the logic of the algorithm without using the specific syntax of a programming language. Pseudo code is an essential tool for algorithm development and analysis because it allows programmers to express their ideas in a simple and understandable way.
Pseudo code typically consists of a set of steps that are written in a structured format using keywords and symbols such as "if-then-else," "for loop," "while loop," and "function." The purpose of pseudo code is to communicate the steps of an algorithm in a clear and concise way, without getting bogged down in the specifics of a particular programming language.
Examples of Pseudo code:
a. Finding the maximum element in an array:
Set max to first element in arrayb. Sorting an array using Selection Sort:
For each element in array:
If the element is greater than max, set max to the element
End for
Return max
For each element in array:
Set minIndex to the current element
For each element after the current element:
If the element is less than the element at minIndex, set minIndex to the index of the new element
End for
If the current element is not equal to the element at minIndex, swap the two elements
End for
3. Data Structures:
Data structures are a way of organizing and storing data in a computer program. They are used to represent and manipulate data efficiently, and to provide a foundation for the development of algorithms. There are various types of data structures, including arrays, linked lists, stacks, queues, trees, and graphs.
Each data structure has its own strengths and weaknesses, and is best suited for a particular set of tasks. For example, arrays are useful for storing a fixed number of elements of the same data type, while linked lists are more flexible and can be used to store a variable number of elements.
Data structures are essential for the development of efficient algorithms because they provide a way to store and access data in a way that is optimized for the task at hand.
Examples of Data Structures:
- a. Arrays: An array is a collection of elements of the same data type. Each element can be accessed by its index.
- b. Linked Lists: A linked list is a collection of nodes, where each node contains a data element and a pointer to the next node in the list.
- c. Stacks: A stack is a data structure that follows the Last-In-First-Out (LIFO) principle. Elements can be added or removed only from the top of the stack.
4. Algorithm Complexity:
Algorithm complexity refers to the amount of time and resources required to run an algorithm. This is typically measured in terms of time complexity and space complexity.
- Time complexity refers to the amount of time required to run an algorithm, as a function of the size of the input. This is typically expressed in terms of the "big O" notation, which provides an upper bound on the growth rate of the algorithm as the input size increases.
- Space complexity refers to the amount of memory required to run an algorithm, as a function of the size of the input. This is also typically expressed in terms of the "big O" notation.
Understanding algorithm complexity is important because it allows programmers to develop algorithms that are efficient and scalable. By minimizing the time and space required to run an algorithm, programmers can create software that can handle large datasets and complex tasks.
Examples of Algorithm Complexity:
- a. Linear Time Complexity: An algorithm that requires a number of operations proportional to the size of the input, such as iterating through an array.
- b. Quadratic Time Complexity: An algorithm that requires a number of operations proportional to the square of the size of the input, such as nested loops used for sorting algorithms.
- c. Constant Space Complexity: An algorithm that requires a fixed amount of memory, such as a simple calculation.
- d. Logarithmic Space Complexity: An algorithm that requires memory proportional to the logarithm of the size of the input, such as a binary search algorithm.
Không có nhận xét nào