[DSA] Mã giả (pseudocode) là gì?
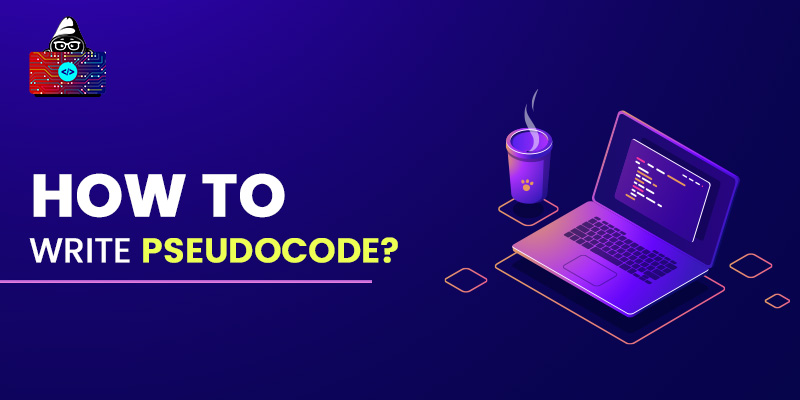
1. Mã giả (Pseudocode) là gì?
Mã giả (Pseudocode) là một ngôn ngữ lập trình giả định
dùng để mô tả thuật toán bằng văn bản có cấu trúc tương tự như các ngôn
ngữ lập trình. Mã giả không phải là ngôn ngữ lập trình cụ thể, mà chỉ
là một cách tiếp cận trừu tượng hóa để giải thích cách thức hoạt động
của thuật toán một cách dễ hiểu cho mọi người, bao gồm cả những người
không có kinh nghiệm lập trình.
Mã giả thường được sử dụng để
diễn tả thuật toán trước khi được chuyển đổi sang ngôn ngữ lập trình cụ
thể như Java, C++, Python... Mã giả giúp cho việc hiểu và phát triển
thuật toán trở nên dễ dàng hơn, vì nó loại bỏ các chi tiết cú pháp và
cấu trúc của ngôn ngữ lập trình, tập trung vào các thuật ngữ toán học và
cấu trúc logic của thuật toán.
Dưới đây là ví dụ về tính tổng các số trong danh sách dùng mã giả:
BEGINSET sum = 0
FOR each number in list
SET sum = sum + number
END FOR
PRINT sum
END
Trong đoạn mã giả trên, chúng ta sử dụng các từ khóa như "SET" để gán giá trị cho biến, "FOR" để bắt đầu một vòng lặp, "END FOR" để kết thúc vòng lặp và "PRINT" để in ra kết quả. Các đoạn mã giả như thế này có thể được sử dụng để trình bày thuật toán một cách dễ hiểu cho những người không quen với các ngôn ngữ lập trình cụ thể.
2. Một số chú ý khi sử dụng mã giả:
Khi sử dụng mã giả (pseudocode), có một số chú ý cần lưu ý để viết mã giả rõ ràng, dễ đọc và dễ hiểu:
- Sử dụng ngôn ngữ và cấu trúc phù hợp: Sử dụng các từ, cấu trúc và ngôn ngữ phù hợp với lĩnh vực và mục đích của thuật toán.
- Định nghĩa rõ ràng các biến và hằng số: Định nghĩa rõ ràng tên và kiểu dữ liệu của các biến và hằng số được sử dụng trong thuật toán.
- Sử dụng các phép toán và cấu trúc điều khiển đúng cách: Sử dụng các phép toán và cấu trúc điều khiển phù hợp và đúng cách để biểu diễn logic của thuật toán.
- Đảm bảo tính đầy đủ và logic của thuật toán: Kiểm tra và đảm bảo tính đầy đủ và logic của thuật toán, đảm bảo thuật toán thực hiện đúng công việc mà nó được thiết kế để thực hiện.
- Sử dụng các chú thích để giải thích các bước của thuật toán: Sử dụng các chú thích và ý kiến để giải thích các bước của thuật toán và làm cho mã giả dễ hiểu hơn.
- Đảm bảo tính linh hoạt của mã giả: Sử dụng mã giả một cách linh hoạt, cho phép dễ dàng thay đổi và cập nhật thuật toán mà không cần phải thay đổi quá nhiều mã giả.
- Sử dụng định dạng và cách thức viết mã giả đồng nhất: Đảm bảo sử dụng cách thức viết mã giả đồng nhất trong toàn bộ thuật toán, để làm cho nó dễ đọc và hiểu hơn.
3. Một số bài tập đơn giản về mã giả:
3.1. Pseudo code to find the sum of two numbers entered by the user:
// Initialize variables to store the two numbers and the sumnum1 = 0
num2 = 0
sum = 0
// Prompt the user to enter the two numbers
print "Enter number 1: "
read num1
print "Enter number 2: "
read num2
// Add the two numbers and store the result in the sum variable
sum = num1 + num2
// Output the result
print "The sum of ", num1, " and ", num2, " is ", sum
3.2. Pseudo code to find the area of a rectangle given the length and width:
// Initialize variables to store the length, width, and area
length = 0, width = 0, area = 0
// Prompt the user to enter the length and width of the rectangle
print "Enter the length of the rectangle: "
read length
print "Enter the width of the rectangle: "
read width
// Calculate the area of the rectangle using the formula length x width
area = length * width
// Output the result
print "The area of the rectangle with length ", length, " and width ", width, " is ", area
Note that in both cases, the pseudo code prompts the user to enter the necessary
3.3. Pseudo code to check if a number entered by the user is even or odd:
// Initialize a variable to store the number entered by the user
number = 0
// Prompt the user to enter a number
print "Enter a number: "
read number
// Check if the number is even or odd using the modulus operator
if number % 2 = 0 then
print number, " is even"
else
print number, " is odd"
end if
3.4. Pseudo code to convert a temperature in Fahrenheit to Celsius:
// Initialize variables to store the temperature in Fahrenheit and Celsius
fahrenheit = 0, celsius = 0
// Prompt the user to enter the temperature in Fahrenheit
print "Enter the temperature in Fahrenheit: "
read fahrenheit
// Convert the temperature to Celsius using the formula (F - 32) * 5/9
celsius = (fahrenheit - 32) * 5/9
// Output the result
print fahrenheit, " degrees Fahrenheit is equal to ", celsius, " degrees Celsius"
3.5. Pseudo code to calculate the factorial of a number entered by the user:
// Initialize variables to store the number entered by the user and the factorial
number = 0, factorial = 1
// Prompt the user to enter a number
print "Enter a number: "
read number
// Calculate the factorial of the number using a loop
for i = 1 to number
factorial = factorial * i
end for
// Output the result
print "The factorial of ", number, " is ", factorial
3.6. Pseudo code to determine if a given year is a leap year or not:
// Initialize a variable to store the year entered by the user
year = 0
// Prompt the user to enter a year
print "Enter a year: "
read year
// Check if the year is a leap year using a conditional statement
if (year % 4 = 0 and year % 100 <> 0) or (year % 400 = 0) then
print year, " is a leap year"
else
print year, " is not a leap year"
end if
Note that in bot
3.7. Pseudo code to sort three numbers entered by the user in ascending order:
// Initialize variables to store the three numbers entered by the user
number1 = 0, number2 = 0, number3 = 0
// Prompt the user to enter three numbers
print "Enter the first number: "
read number1
print "Enter the second number: "
read number2
print "Enter the third number: "
read number3
// Sort the numbers using conditional statements
if number1 <= number2 and number1 <= number3 then
if number2 <= number3 then
print number1, ", ", number2, ", ", number3
else
print number1, ", ", number3, ", ", number2
end if
else if number2 <= number1 and number2 <= number3 then
if number1 <= number3 then
print number2, ", ", number1, ", ", number3
else
print number2, ", ", number3, ", ", number1
end if
else if number3 <= number1 and number3 <= number2 then
if number1 <= number2 then
print number3, ", ", number1, ", ", number2
else
print number3, ", ", number2, ", ", number1
end if
end if
3.8. Pseudo code to convert a binary number entered by the user to decimal:
// Initialize variables to store the binary number entered by the user and the decimal number
binary = 0, decimal = 0, power = 0
// Prompt the user to enter a binary number
print "Enter a binary number: "
read binary
// Convert the binary number to decimal using a loop and the power function
while binary > 0
decimal = decimal + (binary % 10) * (2 ** power)
binary = binary / 10
power = power + 1
end while
// Output the result
print "The decimal equivalent of ", binary, " is ", decimal
3.9. Pseudo code to calculate the average of five numbers entered by the user:
// Initialize variables to store the five numbers entered by the user and their sum
num1 = 0, num2 = 0, num3 = 0, num4 = 0, num5 = 0, sum = 0
// Prompt the user to enter five numbers
print "Enter the first number: "
read num1
print "Enter the second number: "
read num2
print "Enter the third number: "
read num3
print "Enter the fourth number: "
read num4
print "Enter the fifth number: "
read num5
// Calculate the sum of the five numbers
sum = num1 + num2 + num3 + num4 + num5
// Calculate the average of the five numbers
average = sum / 5
// Output the result
print "The average of the five numbers is ", average
3.10. Pseudo code to determine the largest number among three numbers entered by the user:
// Initialize variables to store the three numbers entered by the user
num1 = 0, num2 = 0, num3 = 0, largest = 0
// Prompt the user to enter three numbers
print "Enter the first number: "
read num1
print "Enter the second number: "
read num2
print "Enter the third number: "
read num3
// Determine the largest number using conditional statements
if num1 >= num2 and num1 >= num3 then
largest = num1
else if num2 >= num1 and num2 >= num3 then
largest = num2
else if num3 >= num1 and num3 >= num2 then
largest = num3
end if
// Output the result
print "The largest number is ", largest
4. Một số bài tập thực hành
Write pseudo code to find the sum of the first 10 natural numbers.
Write pseudo code to determine if a given number is positive or negative.
Write pseudo code to convert a given number of minutes to hours and minutes.
Write pseudo code to find the area of a circle given the radius.
Write pseudo code to determine if a given year is a leap year or not (without using any built-in functions).
Write pseudo code to determine if a given string is a palindrome or not.
Write pseudo code to find the largest and smallest numbers in an array of integers.
Write pseudo code to perform linear search on an array of integers to find a given number.
Write pseudo code to find the sum of all odd numbers between 1 and 100 (inclusive).
Write pseudo code to find the factorial of a given number using recursion.
Write pseudo code to determine if a given string is a valid email address.
Write pseudo code to find the second largest number in an array of integers.
Write pseudo code to perform binary search on an array of integers to find a given number.
Write pseudo code to reverse a given string.
Write pseudo code to find the sum of all even numbers between 1 and 100 (inclusive).
Write pseudo code to find the greatest common divisor (GCD) of two numbers.
Write pseudo code to find the area of a triangle given the length of its three sides.
Write pseudo code to convert a given decimal number to binary.
Write pseudo code to determine if a given string is a valid phone number.
Write pseudo code to determine if a given number is prime or not.
Write pseudo code to determine if a given year is a leap year or not (using built-in functions).
Write pseudo code to find the sum of the digits of a given number.
Write pseudo code to determine if a given string is a valid URL.
Write pseudo code to find the median of three numbers entered by the user.
Write pseudo code to find the square root of a given number.
Write pseudo code to find the factorial of a given number using iteration.
Write pseudo code to determine if a given number is a palindrome or not.
Write pseudo code to find the area of a trapezoid given its height and the lengths of its two bases.
Write pseudo code to determine if a given string is a valid password (with specific criteria).
Write pseudo code to find the sum of all numbers between 1 and 100 that are divisible by both 3 and 5
Không có nhận xét nào